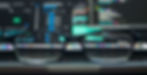
Functional programming is a paradigm that has been gaining popularity in recent years due to its emphasis on writing code that is easier to reason about, test, and maintain. Python, which is a high-level programming language, has a functional programming aspect that developers can use to write clean, efficient, and reusable code.
In this article, we will explore functional programming in Python and how to use iterables, iterators, and generators to create powerful and efficient code.
Iterables
An iterable is a Python object that can be used in a for loop. An iterable object returns an iterator object which is used to iterate over the iterable object. The most common iterable objects in Python are lists, tuples, and strings. However, you can create custom iterable objects by implementing the iter method.
For example, let's create an iterable that returns the first ten even numbers:
class EvenNumbers:
def __init__(self):
self.current = 0
def __iter__(self):
return self
def __next__(self):
self.current += 2
if self.current > 20:
raise StopIteration
return self.current
In the above code, we have created a custom iterable object that returns the first ten even numbers. The iter method returns an iterator object, and the next method returns the next even number until it reaches 20. When it reaches 20, it raises a StopIteration exception to signal the end of the iteration.
Iterators
An iterator is a Python object that returns the next value from an iterable object. An iterator is created by calling the iter method on an iterable object. The next method is used to retrieve the next value from the iterator.
For example, let's use the EvenNumbers iterable we created earlier:
even_numbers = EvenNumbers()
for number in even_numbers:
print(number)
In the above code, we have created an instance of the EvenNumbers iterable object, and we are using a for loop to iterate over the iterable object. The next method is called on the iterator object created by the iter method, and it returns the next even number until it reaches 20.
Generators
A generator is a special type of iterator that allows you to generate a sequence of values on the fly. A generator function is defined using the yield keyword, which returns a value to the caller and temporarily suspends the function's execution. The function can be resumed from where it left off by calling the next() method on the generator object.
For example, let's create a generator that generates the first ten even numbers:
def even_numbers():
current = 0
while True:
current += 2
if current > 20:
return
yield current
In the above code, we have created a generator function that generates the first ten even numbers. The function uses a while loop to generate even numbers until it reaches 20. The yield keyword is used to return the next even number to the caller and temporarily suspend the function's execution.
Coroutines
A coroutine is a function that can pause its execution and return control to its caller, allowing the caller to send data back to the coroutine. A coroutine is defined using the async def keyword, which indicates that the function is a coroutine.
For example, let's create a coroutine that prints the data sent to it:
async def print_data():
while True:
data = await receive_data()
print(data)
In the above code, we have created a coroutine that prints the data sent to it. The coroutine uses the await keyword to receive data from a function named receive_data(). When the coroutine receives data from the receive_data() function, it prints the data to the console. The coroutine can be called using the asyncio module, which provides a framework for asynchronous programming in Python.
itertools
The itertools module in Python provides a collection of functions that can be used to create and manipulate iterators. Some of the commonly used functions in the itertools module are:
count(): Generates an infinite sequence of numbers starting from a given value.
cycle(): Repeats an iterable indefinitely.
repeat(): Repeats an object a specified number of times.
For example, let's use the count() function to generate an infinite sequence of numbers:
from itertools import count
for number in count(start=1, step=2):
if number > 10:
break
print(number)
In the above code, we have used the count() function to generate an infinite sequence of odd numbers starting from 1. We have used a for loop to print the first ten numbers in the sequence.
functools
The functools module in Python provides a collection of higher-order functions that can be used to manipulate functions. Some of the commonly used functions in the functools module are:
reduce(): Applies a function of two arguments cumulatively to the items of an iterable from left to right.
partial(): Returns a new function with some arguments pre-filled.
For example, let's use the reduce() function to calculate the product of a list of numbers:
from functools import reduce
numbers = [1, 2, 3, 4, 5]
product = reduce(lambda x, y: x * y, numbers)
print(product)
In the above code, we have used the reduce() function to calculate the product of a list of numbers using a lambda function.
Operators
The operators module in Python provides a collection of functions that implement the basic operations in Python. Some of the commonly used functions in the operators module are:
add(): Adds two numbers.
mul(): Multiplies two numbers.
itemgetter(): Returns a callable object that retrieves an item from an object.
For example, let's use the itemgetter() function to sort a list of tuples based on the second element:
from operator import itemgetter
students = [('John', 90), ('Peter', 80), ('Mary', 95)] sorted_students = sorted(students, key=itemgetter(1),reverse=True)
print(sorted_students)
In the above code, we have used the itemgetter() function to sort a list of tuples based on the second element (i.e., the marks).
Conclusion
In Python, we can use iterables, iterators, and generators to create powerful and efficient code. We can also use coroutines, itertools, functools, and operators to manipulate functions and data. By understanding these concepts, we can write better code and become more proficient Python developers.